Signal And Slots Qt5
Introduction
Remarks
A slot remains an ordinary function, so you can call it yourself. A single signal can be linked to different slots. A single slot can be called by different linked signals. A connection can be made between a signal and a slot from different objects, and even between objects living inside different threads! Signals and slots are loosely coupled: A class which emits a signal neither knows nor cares which slots receive the signal. Qt's signals and slots mechanism ensures that if you connect a signal to a slot, the slot will be called with the signal's parameters at the right time. Signals and slots can take any number of arguments of any type.
- PyQt5 signals and slots Graphical applications (GUI) are event-driven, unlike console or terminal applications. A users action like clicks a button or selecting an item in a list is called an event. If an event takes place, each PyQt5 widget can emit a signal.
- Qt 5 Documentation — QMainWindow Signals As you can see, alongside the two QMainWindow signals, there are 4 signals inherited from QWidget and 2 signals inherited from Object. If you click through to the QWidget signal documentation you can see a.windowTitleChanged signal implemented here.
- This is a continued tutorial from the previous one, Qt 5 QTcpSocket. We're going to use Signal and Slot mechanism instead of calling functions manually(?). Note: Qt5 document. The QTcpSocket class provides a TCP socket. TCP (Transmission Control Protocol) is a reliable, stream-oriented, connection-oriented transport protocol.
Official documentation on this topic can be found here.
A Small Example
Signals and slots are used for communication between objects. The signals and slots mechanism is a central feature of Qt and probably the part that differs most from the features provided by other frameworks.
The minimal example requires a class with one signal, one slot and one connection:
counter.h
The main
sets a new value. We can check how the slot is called, printing the value.
Finally, our project file:
The new Qt5 connection syntax
The conventional connect
syntax that uses SIGNAL
and SLOT
macros works entirely at runtime, which has two drawbacks: it has some runtime overhead (resulting also in binary size overhead), and there's no compile-time correctness checking. The new syntax addresses both issues. Before checking the syntax in an example, we'd better know what happens in particular.
Let's say we are building a house and we want to connect the cables. This is exactly what connect function does. Signals and slots are the ones needing this connection. The point is if you do one connection, you need to be careful about the further overlaping connections. Whenever you connect a signal to a slot, you are trying to tell the compiler that whenever the signal was emitted, simply invoke the slot function. This is what exactly happens.
Here's a sample main.cpp:
Hint: the old syntax (SIGNAL
/SLOT
macros) requires that the Qt metacompiler (MOC) is run for any class that has either slots or signals. From the coding standpoint that means that such classes need to have the Q_OBJECT
macro (which indicates the necessity to run MOC on this class).
The new syntax, on the other hand, still requires MOC for signals to work, but not for slots. If a class only has slots and no signals, it need not have the Q_OBJECT
macro and hence may not invoke the MOC, which not only reduces the final binary size but also reduces compilation time (no MOC call and no subsequent compiler call for the generated *_moc.cpp
file).
Connecting overloaded signals/slots
While being better in many regards, the new connection syntax in Qt5 has one big weakness: Connecting overloaded signals and slots. In order to let the compiler resolve the overloads we need to use static_cast
s to member function pointers, or (starting in Qt 5.7) qOverload
and friends:
Multi window signal slot connection
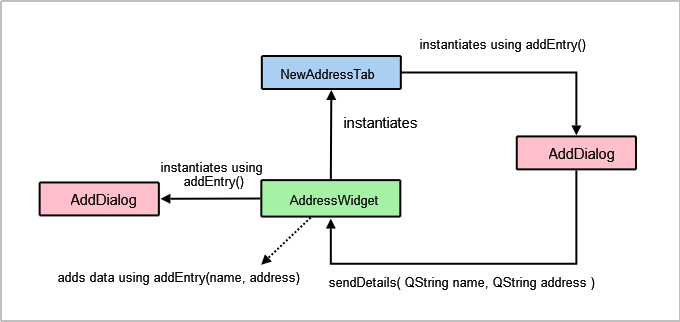
A simple multiwindow example using signals and slots.
There is a MainWindow class that controls the Main Window view. A second window controlled by Website class.
The two classes are connected so that when you click a button on the Website window something happens in the MainWindow (a text label is changed).
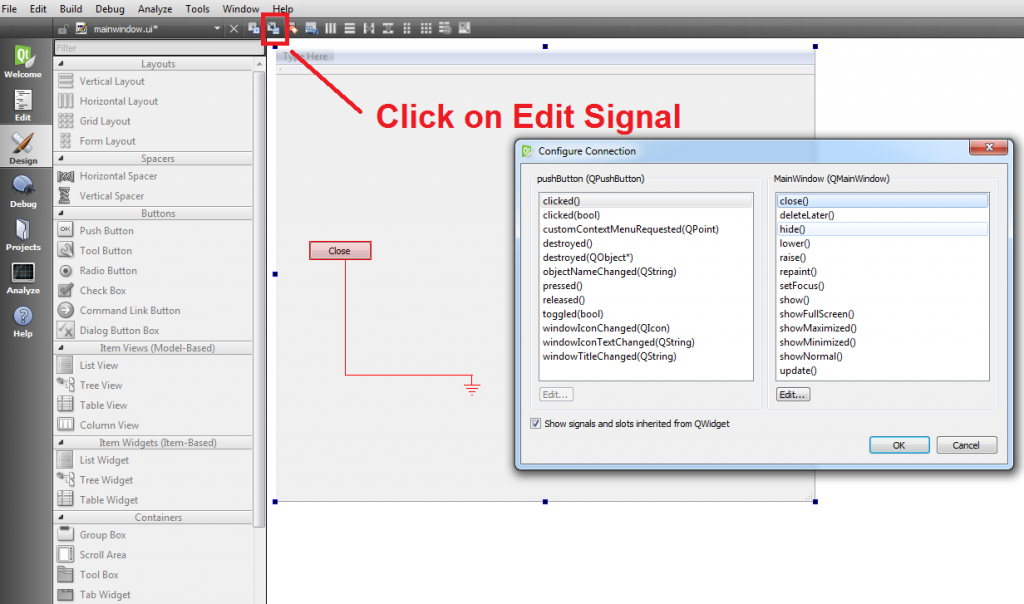
I made a simple example that is also on GitHub:
mainwindow.h
mainwindow.cpp
website.h
website.cpp
Project composition:
Consider the Uis to be composed:
- Main Window: a label called 'text' and a button called 'openButton'
- Website Window: a button called 'changeButton'
So the keypoints are the connections between signals and slots and the management of windows pointers or references.
Build complex application behaviours using signals and slots, and override widget event handling with custom events.
As already described, every interaction the user has with a Qt application causes an Event. There are multiple types of event, each representing a difference type of interaction — e.g. mouse or keyboard events.
Events that occur are passed to the event-specific handler on the widget where the interaction occurred. For example, clicking on a widget will cause a QMouseEvent
to be sent to the .mousePressEvent
event handler on the widget. This handler can interrogate the event to find out information, such as what triggered the event and where specifically it occurred.
You can intercept events by subclassing and overriding the handler function on the class, as you would for any other function. You can choose to filter, modify, or ignore events, passing them through to the normal handler for the event by calling the parent class function with super()
.
However, imagine you want to catch an event on 20 different buttons. Subclassing like this now becomes an incredibly tedious way of catching, interpreting and handling these events.
Thankfully Qt offers a neater approach to receiving notification of things happening in your application: Signals.
Signals
Instead of intercepting raw events, signals allow you to 'listen' for notifications of specific occurrences within your application. While these can be similar to events — a click on a button — they can also be more nuanced — updated text in a box. Data can also be sent alongside a signal - so as well as being notified of the updated text you can also receive it.
The receivers of signals are called Slots in Qt terminology. A number of standard slots are provided on Qt classes to allow you to wire together different parts of your application. However, you can also use any Python function as a slot, and therefore receive the message yourself.
Load up a fresh copy of `MyApp_window.py` and save it under a new name for this section. The code is copied below if you don't have it yet.
Basic signals
First, let's look at the signals available for our QMainWindow
. You can find this information in the Qt documentation. Scroll down to the Signals section to see the signals implemented for this class.
Qt 5 Documentation — QMainWindow Signals
As you can see, alongside the two QMainWindow
signals, there are 4 signals inherited from QWidget
and 2 signals inherited from Object
. If you click through to the QWidget
signal documentation you can see a .windowTitleChanged
signal implemented here. Next we'll demonstrate that signal within our application.
Qt 5 Documentation — Widget Signals
The code below gives a few examples of using the windowTitleChanged
signal.
Try commenting out the different signals and seeing the effect on what the slot prints.
We start by creating a function that will behave as a ‘slot’ for our signals.
Then we use .connect on the .windowTitleChanged
signal. We pass the function that we want to be called with the signal data. In this case the signal sends a string, containing the new window title.
If we run that, we see that we receive the notification that the window title has changed.
Events
Next, let’s take a quick look at events. Thanks to signals, for most purposes you can happily avoid using events in Qt, but it’s important to understand how they work for when they are necessary.
As an example, we're going to intercept the .contextMenuEvent
on QMainWindow
. This event is fired whenever a context menu is about to be shown, and is passed a single value event
of type QContextMenuEvent
.
To intercept the event, we simply override the object method with our new method of the same name. So in this case we can create a method on our MainWindow
subclass with the name contextMenuEvent
and it will receive all events of this type.
Signal And Slot Qt C++
If you add the above method to your MainWindow
class and run your program you will discover that right-clicking in your window now displays the message in the print statement.
Sometimes you may wish to intercept an event, yet still trigger the default (parent) event handler. You can do this by calling the event handler on the parent class using super
as normal for Python class methods.
This allows you to propagate events up the object hierarchy, handling only those parts of an event handler that you wish.
However, in Qt there is another type of event hierarchy, constructed around the UI relationships. Widgets that are added to a layout, within another widget, may opt to pass their events to their UI parent. In complex widgets with multiple sub-elements this can allow for delegation of event handling to the containing widget for certain events.
However, if you have dealt with an event and do not want it to propagate in this way you can flag this by calling .accept()
on the event.
Alternatively, if you do want it to propagate calling .ignore()
will achieve this.
Signals And Slots Qt5
In this section we've covered signals, slots and events. We've demonstratedsome simple signals, including how to pass less and more data using lambdas.We've created custom signals, and shown how to intercept events, pass onevent handling and use .accept()
and .ignore()
to hide/show eventsto the UI-parent widget. In the next section we will go on to takea look at two common features of the GUI — toolbars and menus.